With the rapidly changing technologies, developers are being provided with incredible new tools and APIs. But it has been seen that out of the 100+ APIs, only 5% of them are actively used by developers.
Let’s take a look at some of the useful Web APIs that can help you skyrocket your website to the moon! 🌕🚀
1. Screen Capture API
The Screen Capture API, as the name suggests, allows you to capture the contents of a screen, making the process of building a screen recorder a piece of cake.
You need a video element to display the captured screen. The start button will start the screen capture.
<video id="preview" autoplay>
Your browser doesn't support HTML5.
</video>
<button id="start" class="btn">Start</button>
const previewElem = document.getElementById("preview");
const startBtn = document.getElementById("start");
async function startRecording() {
previewElem.srcObject =
await navigator.mediaDevices.getDisplayMedia({
video: true,
audio: true,
});
}
startBtn.addEventListener("click", startRecording);
2. Web Share API
The Web Share API allows you to share text, links, and even files from a web page to other apps installed on the device.
async function shareHandler() {
navigator.share({
title: "Tapajyoti Bose | Portfolio",
text: "Check out my website",
url: "https://tapajyoti-bose.vercel.app/",
});
}
NOTE: To use the Web Share API, you need an interaction from the user. For example, a button click or a touch event.
3. Intersection Observer API
The Intersection Observer API allows you to detect when an element enters or leaves the viewport. This is exceptionally useful for implementing infinite scroll.
NOTE: The demo uses React because of my personal preference, but you can use any framework or vanilla JavaScript.
4. Clipboard API
The Clipboard API allows you to read and write data to the clipboard. This is useful for implementing the copy to clipboard functionality.
async function copyHandler() {
const text = "https://tapajyoti-bose.vercel.app/";
navigator.clipboard.writeText(text);
}
5. Screen Wake Lock API
Ever wondered how YouTube prevents the screen from being switched off while playing the video? Well, that’s because of the Screen Wake Lock API.
let wakeLock = null;
async function lockHandler() {
wakeLock = await navigator.wakeLock.request("screen");
}
async function releaseHandler() {
await wakeLock.release();
wakeLock = null;
}j
NOTE: You can only use the Screen Wake Lock API if the page is already visible on the screen. Otherwise, it would throw an error.
6. Screen Orientation API
The Screen Orientation API allows you to check the current orientation of the screen and even lock it to a specific orientation.
async function lockHandler() {
await screen.orientation.lock("portrait");
}
function releaseHandler() {
screen.orientation.unlock();
}
function getOrientation() {
return screen.orientation.type;
}
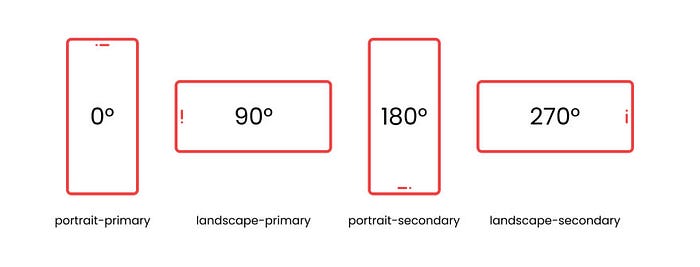
7. Fullscreen API
The Fullscreen API allows you to display an element or the entire page in full screen.
async function enterFullscreen() {
await document.documentElement.requestFullscreen();
}
async function exitFullscreen() {
await document.exitFullscreen();
}
NOTE: To use the Fullscreen API too, you need an interaction from the user.
'프로그래밍 > Script' 카테고리의 다른 글
[jQuery] scrollHeight 가져오기 (0) | 2023.07.07 |
---|---|
[jQuery] URL에서 해시 값 가져오기 (0) | 2023.07.04 |
[script] 입력창 글자수 제한 (0) | 2023.07.03 |
jquery keyup, keydown, keypress 차이 (0) | 2023.07.03 |
document.ready() vs window.load() 차이 (0) | 2023.07.03 |