How to Implement Deep Linking in Flutter?
Flutter에서 딥링킹을 구현하는 방법은 무엇입니까?
https://medium.com/@dudhatkirtan/how-to-implement-deep-linking-in-flutter-f882b6d834da
How to Implement Deep Linking in Flutter?
Overview
medium.com
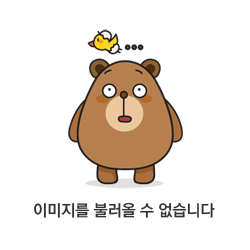
Overview
Deeplink in Flutter means the ability to open a specific page or perform a specific action within your app by clicking on a link from another app or a web page. It allows users to navigate to specific pages or sections of your app like cart, orders, or any product information, etc. Deeplink in Flutter is a very good feature for all developers and users. Flutter supports deep linking on iOS, Android, and web browsers. Implementing deep linking in Flutter apps enhances the user experience and makes it easier for users to navigate directly to specific sections.
Introduction
Deep linking is a crucial feature in mobile app development that enables smooth navigation to specific sections of different apps. This is very useful for users to access relevant content or perform specific tasks without manually navigating through the app’s screens. This deep linking feature eliminates the need for users to search for or manually locate the desired information within the app. Deep linking is a useful functionality for apps like e-commerce apps, content-driven apps, and social media platforms. By using deep links in Flutter, we can connect with other apps and services making it a good digital ecosystem.
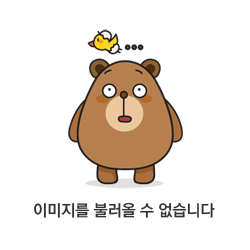
Understanding Deep Links in Flutter
DeepLinks in Flutter means you have URLs or URIs to navigate or perform specific actions within a Flutter app. It directly helps users to access specific screens or content like webpages, screens, and notifications.
There are some concepts and terms that should be known to developers for using deep links in the Flutter applications.
- URL Scheme -
- A URL scheme is a unique identifier that identifies your app and allows it to handle specific deep links. It is generally a link to view or see a particular product in an e-commerce app.
Universal Links (iOS) and App Links (Android):
- These are platform-specific mechanisms that help deep linking to work smoothly between web content and apps. These help in directly opening the content of the app, if the app is installed on the device, or fall back to the web page if the app is not present.
Deep Link Handling :
- We need to handle the logic for deep lonks in Flutter like capturing the link URL and getting important data such as parameters or query strings. This captured information helps in navigating to a particular screen or performing the actions.
Implement Deep Linking on Android
To use deep links in your Flutter applications, we must have links for redirection that can be instantiated by adding intent filters for the incoming links.
In Android, we have two types of Uni Links, which are approximately the same as the meaning.
Open the DynamicLink section in firebase
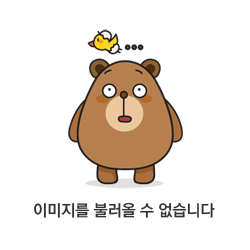
- On a domain that you may customize by adding your own name, company name, trademark, etc., dynamic links are generated. More relevant links that have been customized appear. After displaying this window, click Finish.
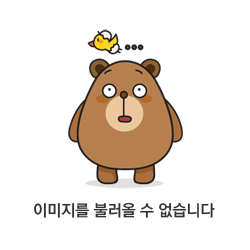
- Now, on the newly opened page, select the “New Dynamic link” button.
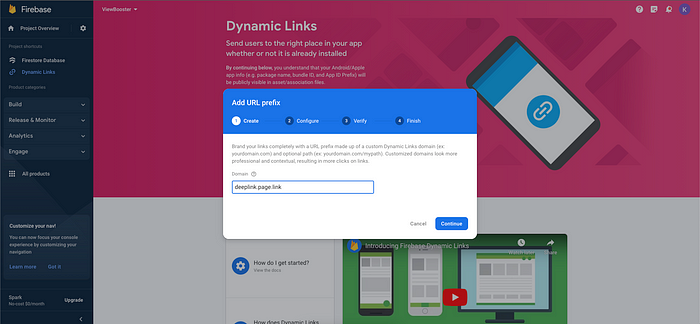
- Click on the new dynamic link button.
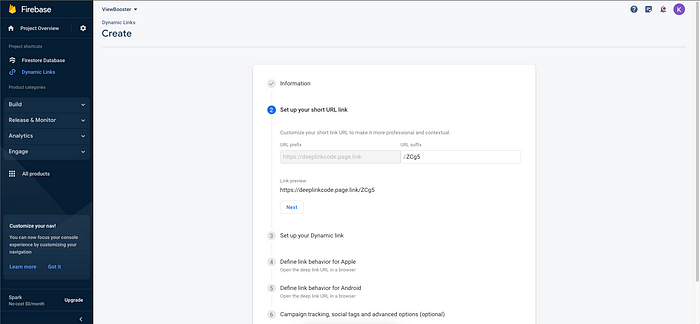
App Links -
App Links are the same as deep links, but it does require a specified host, i.e. a hosted file and along with this, the app links only tend to work with the HTTPS scheme.
You need to add the following code to your AndroidManifest file for adding deep links in Flutter. There you have to define an intent-filter and you can change the host with your need.
<!-- This is for App Links -->
<meta-data
android:name="flutter_deeplinking_enabled"
android:value="true" />
<intent-filter android:autoVerify="true">
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- Accepts URIs that begin with https://YOUR_HOST -->
<data
android:scheme="http"
android:host="deeplink.example.com" />
<data android:scheme="https" />
</intent-filter>
Deep Links -
Deep links are the links in Android, which don’t require a specified host or any other custom scheme.
As discussed above, we have to paste for Deep links and we can change the host and scheme accordingly.
<!-- This is for Deep Links -->
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- Accepts URIs that begin with YOUR_SCHEME://YOUR_HOST -->
<data android:scheme="blog" android:host="deeplink.example" />
</intent-filter>
We can use any of the intent filters in the AndroidManifest.xml file.
The intent filter is a statement in the manifest file that specifies what intent or component will be received on the app side, according to native app implementation. When an action occurs, it can specify additional actions that should be taken on this intent filter.
Data is what the URI format, which primarily resolves to the activity, represents. Also, a data tag must have the android attribute.
A category is mainly the type of intent-filter category. We have defined BROWSABLE in the category, which means that the intent filter can be accessed from the browser, and if not added, it will not be browsed.
Implement Deep Linking on iOS
In iOS also, we have two types of links-
Universal Links :
Universal links are those links that require a specified host or a custom scheme in their app. It works only with the HTTPS scheme. Below is the defined example of a universal link.
For setting up Universal Links we need to follow some steps-
- Enable Associated Domains in your app’s entitlements.
- Set up a unique URL identifier for your app.
- Set up a JSON file on your website that contains information about your app’s URL identifier.
Enable Associated Domains
Creating the entitlements file in Xcode:
- Open up Xcode by double-clicking on your ios/Runner.xcworkspace file.
- Go to the Project navigator (Cmd+1) and select the Runner root item at the very top.
- Select the Runner target and then the Signing & Capabilities tab.
- Click the + Capability (plus) button to add a new capability.
- Type “associated domains” and select the item.
- Double-click the first item in the Domains list and change it from webcredentials.com to: applinks: + your host (ex: my-blogs.com). A file called Runner.entitlements will be created and added to the project.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<!-- ... other keys -->
<key>com.apple.developer.associated-domains</key>
<array>
<string>applinks:[YOUR_HOST_HERE]</string>
</array>
<!-- ... other keys -->
</dict>
</plist>
Custom URL:
In Custom URL, we don’t need a host or a custom scheme for our iOS app. An example of a custom URL is defined below. We need to add the host and scheme in the ios/Runner/Info.plist file.
<key>FlutterDeepLinkingEnabled</key>
<true/>
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleTypeRole</key>
<string>Editor</string>
<key>CFBundleURLName</key>
<string>flutterbooksample.com</string>
<key>CFBundleURLSchemes</key>
<array>
<string>customscheme</string>
</array>
</dict>
</array>
Handling Deep Linking in the App
There are two ways your app will receive a link — from a cold start and brought from the background.
ATTENTION -
getInitialLink/getInitialUri should be handled ONLY ONCE in your app’s lifetime, since it is not meant to change throughout your app’s life
Once we are done with the Universal Links set up for our app, we will need to handle the links within our Flutter app. To do this, we need to use the uni_links package. Add this dependency in pubspec.yaml file and pub get it.
uni_links: ^0.5.1
Now, we just need to register a callback to handle incoming deep links.
import 'package:uni_links/uni_links.dart';
void main() {
// Initialize the deep link handler
initUniLinks();
runApp(MyApp());
}
void initUniLinks() async {
// Ensure that the app is ready to handle deep links
await getInitialUri();
// Set up a stream subscription to handle deep links when the app is running
uriSubscription = uriLinkStream.listen((Uri uri) {
// Handle the deep link here
handleDeepLink(uri);
});
}
void handleDeepLink(Uri uri) {
// Process the deep link URI and navigate accordingly
// You can extract parameters or perform specific actions based on the deep link data
}
Advanced Usage
Some additional features enhance the capabilities of deep linking in our Flutter app. These features help in creating more personalized and smooth user experiences, tracking user engagement, optimizing marketing efforts, and improving overall app performance.
Deep Link Analytics -
By implementing Deep Link Analytics, we can track and analyze user engagement with deep links. We can get the data on the number of clicks, conversions, and user behavior after accessing the app through a deep link. With this information, we can get to know about our deep links and can do some optimization if needed.
Deferred Deep Linking -
This helps in handling the scenarios, where the app is not installed on the user’s device on clicking the deep link. In these cases, the user is redirected to the app store to download the app and after installation, the app can open and handle the deep link that triggered the installation.
Dynamic Deep Links -
These help in generating deep links programmatically with dynamic parameters. It means we can generate deep links that include user-specific data, product IDs, or other variables.
Deep Link Attribution -
By using this you can track the source of each deep link and can know which channels are getting more engagement and conversions.
Deferred App Deep Linking -
Some platforms handle deep links when the app is already installed but not in the foreground. But this feature works even if the app is in the background or not currently running.
Example App
Let us understand this through a code. In the AndroidManifest.xml file, I have declared my link like — blog://deeplink.example
We will declare the function main.dart file like this.
import 'package:blog/home.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:uni_links/uni_links.dart';
import 'package:flutter/services.dart' show PlatformException;
void main() {
initUniLinks();
runApp(MyApp());
initUniLinks()async{
try{
Uri? initialLink = await getInitialUri();
print(initialLink);
} on PlatformException {
print('platfrom exception unilink');
}
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context){
return MaterialApp(
home: HomeScreen(),
);
}
}
There is some page that says it as HomeScreen.
import 'package:flutter/material.dart';
class HomeScreen extends StatefulWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
@override
Widget build(BuildContext context) {
return const Scaffold(
body: Center(
child: Text("Home Screen"),
),
);
}
}
We will open this app through the deeplink shown below. We will paste the same link(blog://deeplink.example) in the browser and it will show us the APP NAME of that app and will navigate us to the app by clicking on continue
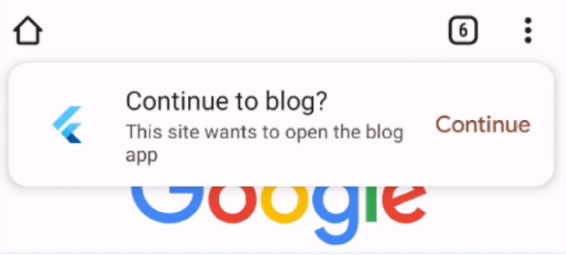
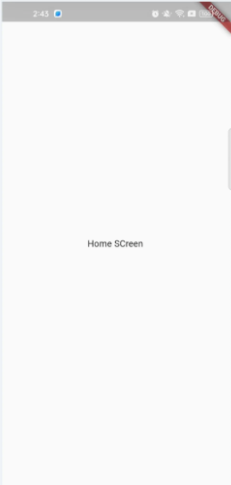
Conclusion
- Deep links help in seamless navigation to specific screens from external sources like the web, notifications, etc.
- By using deep links, users are more engaged and satisfied. This also helps users to users to access relevant and personalized content.
- Deep links provide cross-platform compatibility like for both iOS and Android.
- Deep links can be customized with parameters or query strings, allowing for dynamic and personalized experiences based on user-specific data.
- We can test and analyze deep links, also we can track user behavior, and optimize our app’s deep linking strategy.
- By using deep links in Flutter, we can interact with other apps, services, or platforms. Also, we can collaborate and share the data.
'프로그래밍 > App' 카테고리의 다른 글
[Flutter] Flutter AI 통합: 모바일 앱 재정의 (0) | 2023.11.24 |
---|---|
Flutter vs. React Native (0) | 2023.11.16 |
[Flutter] Best Flutter IDE for Front-End Development (0) | 2023.11.01 |
YouTube Music은 Android 및 iOS에서 더 많은 사용자에게 실시간 가사를 제공합니다. (0) | 2023.08.29 |
Flutter and Clean Architecture(I) (0) | 2023.08.29 |