반응형
pip install wifi-qrcode-generator
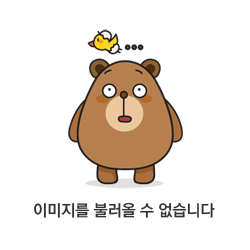
Python을 사용하여 Wi-Fi QR 코드를 생성하려면 qrcode 및 Pillow 라이브러리를 활용할 수 있습니다. Wi-Fi QR 코드의 데이터 형식은 다음과 같습니다:
import qrcode
from PIL import Image
def generate_wifi_qrcode(ssid, password, encryption="WPA", hidden=False, output_file="wifi_qrcode.png"):
"""
Generates a Wi-Fi QR code and saves it as an image.
:param ssid: The Wi-Fi network name (SSID)
:param password: The Wi-Fi password
:param encryption: Encryption type ('WPA', 'WEP', or 'nopass')
:param hidden: Whether the network is hidden (True/False)
:param output_file: Name of the output image file
"""
# Format Wi-Fi QR code data
wifi_data = f"WIFI:S:{ssid};T:{encryption};P:{password};H:{'true' if hidden else 'false'};;"
# Generate QR code
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
qr.add_data(wifi_data)
qr.make(fit=True)
# Create and save the QR code image
img = qr.make_image(fill_color="black", back_color="white")
img.save(output_file)
print(f"Wi-Fi QR code saved as {output_file}")
# Open and show the image
img.show()
# Example usage
generate_wifi_qrcode(
ssid="MyWiFiNetwork",
password="MySecurePassword",
encryption="WPA", # WPA/WEP/nopass
hidden=False, # Hidden network? True/False
output_file="wifi_qrcode.png"
)
코드 설명
- Wi-Fi QR 코드 형식:
- WIFI:S:<SSID>;T:<WPA/WEP/nopass>;P:<Password>;H:<Hidden (true/false)>;;
- S: Wi-Fi 이름 (SSID)
- T: 암호화 방식 (WPA, WEP, nopass)
- P: 비밀번호
- H: 네트워크 숨김 여부 (true 또는 false)
- WIFI:S:<SSID>;T:<WPA/WEP/nopass>;P:<Password>;H:<Hidden (true/false)>;;
- QR 코드 생성:
- **qrcode.QRCode**를 사용하여 데이터를 추가하고 이미지를 생성.
- QR 코드는 기본적으로 검은색 fill_color와 흰색 back_color를 사용하여 생성.
- 결과 저장 및 표시:
- 생성된 QR 코드를 wifi_qrcode.png로 저장.
- **img.show()**를 사용하여 시스템 기본 이미지 뷰어에서 표시.
결과
- wifi_qrcode.png 파일이 생성됩니다.
- QR 코드를 스캔하면 자동으로 Wi-Fi 네트워크에 연결할 수 있습니다.
wifi_qrcode_generator
Generate a QR code for your WiFi network to let others quickly connect without needing to tell them your long and complicated password.
Installation
$ pip install wifi-qrcode-generator
Usage
CLI interactive mode
$ wifi-qrcode-generator
CLI non-interactive mode
$ wifi-qrcode-generator -s "Home WiFi" -p "very complicated password" -a WPA -o qr.png -P
Python API
#!/usr/bin/env python3
import wifi_qrcode_generator.generator
qr_code = wifi_qrcode_generator.generator.wifi_qrcode(
ssid='Home WiFi', hidden=False, authentication_type='WPA', password='very complicated password'
)
qr_code.print_ascii()
qr_code.make_image().save('qr.png')
Dependencies
- Pillow
- qrcode
wifi_qrcode_generator
Generate a QR code for your WiFi network to let others quickly connect without needing to tell them your long and complicated password.
Usage$ pip install wifi-qrcode-generator
$ wifi-qrcode-generator
Dependencies
- Pillow
- qrcode
wifi_qrcode_generator
Generate a QR code for your WiFi network to let others quickly connect without needing to tell them your long and complicated password.
Usage$ pip install wifi-qrcode-generator
$ wifi-qrcode-generator
Dependencies
- #!/usr/bin/env python3 import wifi_qrcode_generator.generator qr_code = wifi_qrcode_generator.generator.wifi_qrcode( ssid='Home WiFi', hidden=False, authentication_type='WPA', password='very complicated password' ) qr_code.print_ascii() qr_code.make_image().save('qr.png')
- Python API
- $ wifi-qrcode-generator -s "Home WiFi" -p "very complicated password" -a WPA -o qr.png -P
- CLI non-interactive mode
- CLI interactive mode
- Installation
- #!/usr/bin/env python3 import wifi_qrcode_generator.generator qr_code = wifi_qrcode_generator.generator.wifi_qrcode( ssid='Home WiFi', hidden=False, authentication_type='WPA', password='very complicated password' ) qr_code.print_ascii() qr_code.make_image().save('qr.png')
- Python API
- $ wifi-qrcode-generator -s "Home WiFi" -p "very complicated password" -a WPA -o qr.png -P
- CLI non-interactive mode
- CLI interactive mode
- Installation
https://pypi.org/project/wifi-qrcode-generator/
wifi-qrcode-generator
Generate a QR code for your WiFi network to let others quickly connect.
pypi.org
반응형
'프로그래밍 > Python' 카테고리의 다른 글
[python] Spiral Web using Matplotlib and NumPy (0) | 2024.12.03 |
---|---|
[python] 초를 입력 받으면 카운트다운 하는 간단한 타이머 (0) | 2024.12.02 |
[python] Sunburst Chart (0) | 2024.11.26 |
[python] What’s New in Python 3.13 (0) | 2024.11.19 |
[python] pyQt, requests, BeautifulSoup 이용한 웹크롤링 (1) | 2024.11.15 |